JavaScript chart rendering involves several steps: data fetching, processing, and the actual rendering of the chart. Each of these steps can introduce performance bottlenecks. Before diving into optimization techniques, it’s essential to understand the fundamental processes involved:
Data Fetching
It is the initial step where information is retrieved from a server or a local source. The speed of this process can be influenced by factors such as network latency, size, and server performance. Efficient fetching is crucial for minimizing initial load times.
Data Processing
Once the information is fetched, it often needs to be processed or transformed to fit the requirements of the charting library. This step might involve filtering, aggregating, or formatting. Inefficient processing can lead to significant delays before the rendering even begins.
Rendering
The final step is the actual rendering of the chart. This involves using a charting library such as SciChart, which can be found at https://www.scichart.com/javascript-chart-features/, to draw the chart on the web page. The rendering performance depends on the complexity of the chart, the number of points, and the capabilities of the browser.
Optimizing Data Fetching
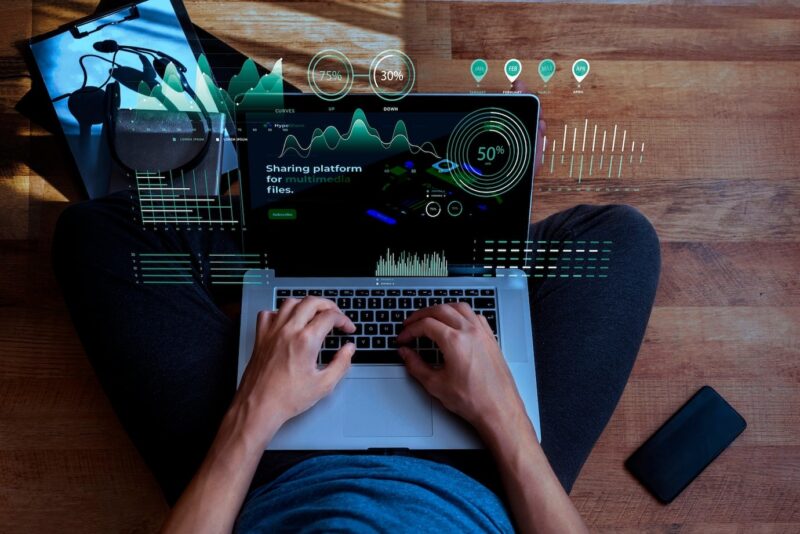
Reducing Size
One of the simplest ways to improve fetching performance is to reduce the size of the information being fetched. This can be achieved by implementing server-side filtering and aggregation. By only fetching the necessary details, the amount of time spent transferring over the network can be significantly reduced.
Compression
Compressing information before transmission can also help speed up fetching. Techniques such as Gzip compression can reduce the size of the payload, resulting in faster download times. Most modern web servers support compression, and enabling it can lead to substantial performance gains.
Asynchronous Loading
Using asynchronous loading techniques such as AJAX or Fetch API allows the rest of the web page to load while the information is being fetched. This can improve the perceived performance of the application, as users can start interacting with the page even before the chart data is fully loaded.
Enhancing Data Processing
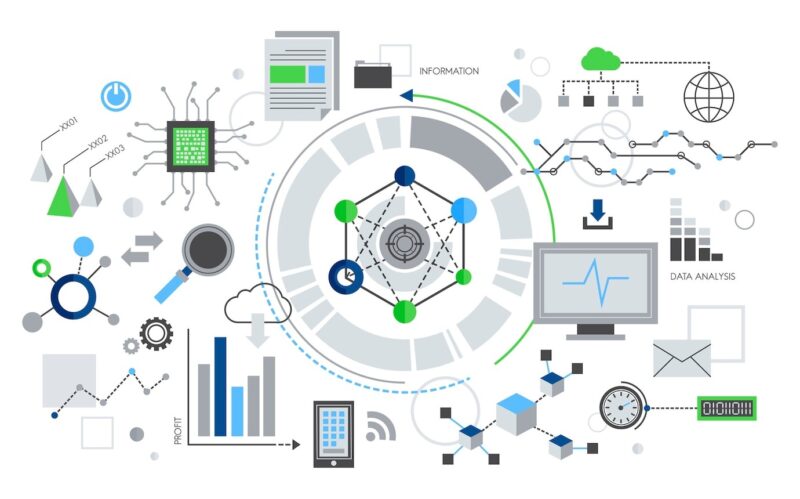
Efficient Structures
Choosing the right structures can have a significant impact on processing performance. For example, using arrays for simple collections and objects or maps for key-value pairs can optimize the performance of common operations like searching, filtering, and aggregating.
Web Workers
Web Workers provide a way to run scripts in background threads, separate from the main execution thread of a web application. By offloading processing tasks to Web Workers, the main thread remains responsive, leading to smoother user interactions and faster rendering times.
Lazy Loading and Pagination
For extremely large sets, it might be impractical to process everything at once. Implementing lazy loading or pagination can help manage information in smaller, more manageable chunks. This not only reduces the initial processing load but also allows users to interact with the data incrementally.
Optimizing Chart Rendering
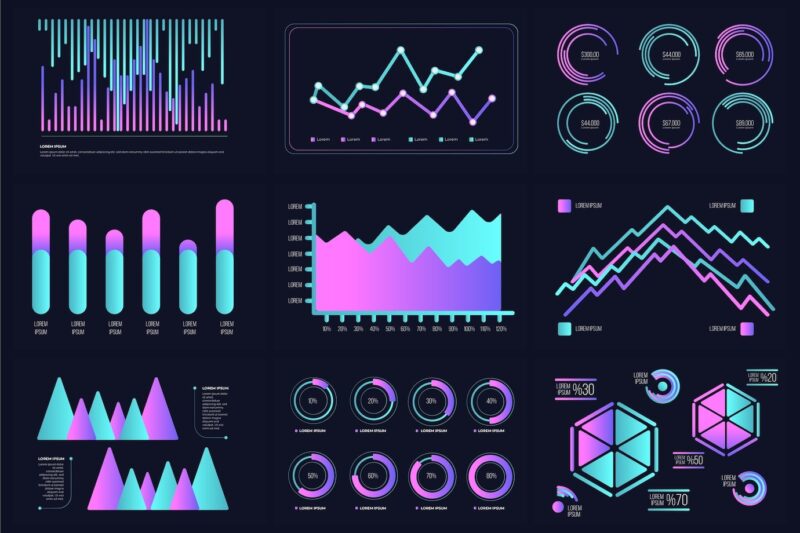
Choosing the Right Charting Library
Not all charting libraries are created equal. Some are optimized for performance and can handle large sets more efficiently than others. When selecting a charting library, consider factors such as the number of points it can handle, the types of charts it supports, and its overall performance characteristics.
Simplifying Chart Complexity
Complex charts with numerous points, intricate animations, and multiple layers can significantly slow down rendering performance. Simplifying the chart by reducing the number of points, minimizing the use of animations, and limiting the number of chart layers can help improve rendering speeds.
Canvas vs. SVG
JavaScript charting libraries typically use either the Canvas API or SVG (Scalable Vector Graphics) for rendering. Each has its strengths and weaknesses. Canvas is generally faster for rendering large numbers of simple shapes, making it suitable for data-intensive charts.
SVG, on the other hand, provides better scalability and quality for complex charts with fewer points. Choosing the appropriate rendering method based on the specific requirements of the chart can lead to performance improvements.
Hardware Acceleration
Modern browsers support hardware acceleration, which can offload rendering tasks to the GPU (Graphics Processing Unit) instead of the CPU. Enabling hardware acceleration can significantly improve rendering performance, especially for complex and interactive charts.
Advanced Techniques for Boosting Performance
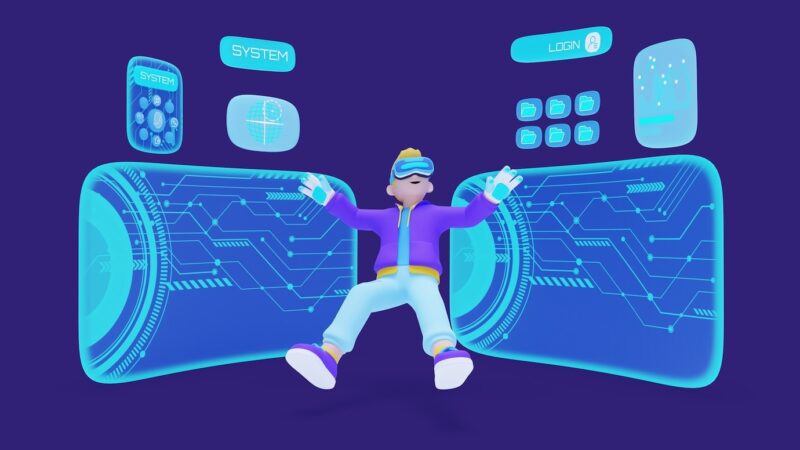
Virtualization
Virtualization involves rendering only the visible portion of the chart and dynamically updating it as the user scrolls or interacts with the chart. This technique can be particularly effective for handling large sets, as it reduces the rendering load by focusing only on the information that is currently in view.
Pre-Rendering and Caching
Pre-rendering charts on the server side and caching the rendered output can dramatically reduce the load on the client side. When a user requests a chart, the pre-rendered version can be quickly served from the cache, bypassing the need for client-side rendering. This approach is especially useful for static or infrequently changing charts.
Throttling and Debouncing
Throttling and debouncing are techniques used to limit the frequency of function executions. In the context of chart rendering, they can be used to control how often the chart is updated in response to user interactions, such as resizing the window or zooming in/out. By reducing the number of updates, these techniques help maintain smooth performance.
Progressive Rendering
Progressive rendering involves rendering the chart in stages rather than all at once. This can create a smoother user experience by quickly displaying a simplified version of the chart and gradually enhancing it with more details. This technique can be particularly effective for large and complex charts, as it provides immediate visual feedback to the user.
Real-World Examples and Case Studies
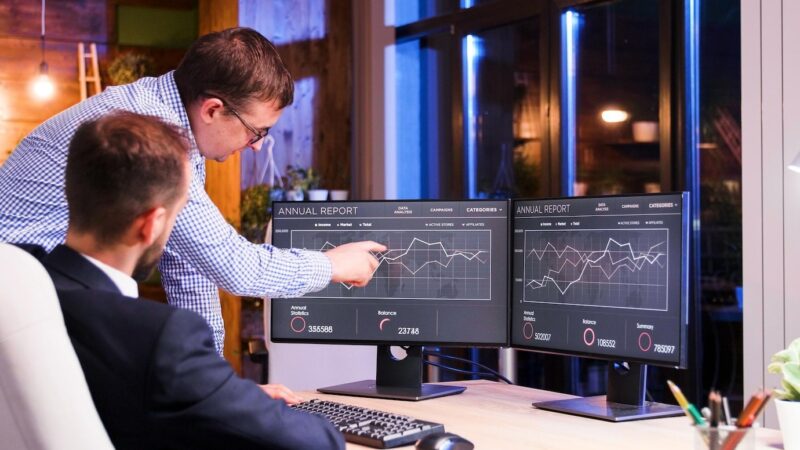
1. Financial Data Visualization
A financial services company needed to display large volumes of historical stock price information in real-time. Initially, the charts were sluggish and unresponsive due to the sheer volume of points. By implementing server-side aggregation, the size was reduced before transmission.
Web Workers were used to process the information in the background, and a Canvas-based charting library was chosen for its performance advantages with large sets. These optimizations resulted in a significant improvement in rendering speed, allowing users to interact with the charts seamlessly.
2. E-Commerce Sales Dashboard
An e-commerce platform required a dashboard to visualize sales information from thousands of transactions. The initial implementation suffered from slow load times and laggy interactions. To address these issues, the information was compressed before transmission, and asynchronous loading was implemented.
Lazy loading was used to handle large sets, and a virtualized charting library was chosen to render only the visible portion of the chart. These changes led to faster load times and smoother interactions, enhancing the overall user experience.
3. IoT Data Monitoring
An IoT company needed to monitor information from thousands of sensors in real-time. The initial charts were overwhelmed by the volume of incoming data, leading to slow and unresponsive visualizations.
By enabling hardware acceleration and implementing throttling to control the frequency of updates, the rendering performance was significantly improved. Additionally, a progressive rendering approach was used to provide immediate visual feedback, ensuring that users could quickly interpret the data.
Future Trends in JavaScript Chart Rendering
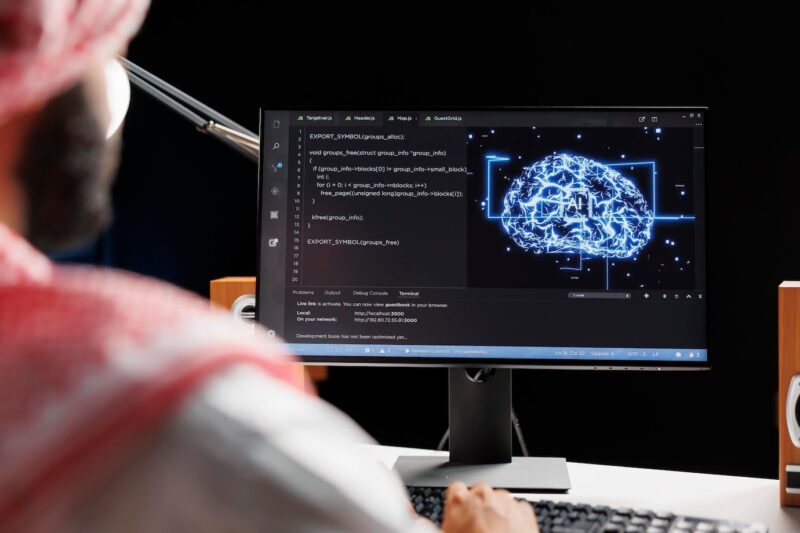
As technology continues to evolve, new trends and innovations are emerging in the field of JavaScript chart rendering. Staying abreast of these developments can help developers create even more efficient and responsive visualizations.
WebAssembly
WebAssembly is a binary instruction format that allows code written in multiple languages to run on the web at near-native speed. By leveraging WebAssembly, developers can offload computationally intensive tasks to more efficient languages like C or Rust, resulting in faster processing and rendering.
Machine Learning
Machine learning algorithms can be used to optimize various aspects of chart rendering, such as predicting user interactions and pre-fetching information. By incorporating machine learning, developers can create smarter and more responsive visualizations that adapt to user behavior.
Real-Time Data Streaming
As the demand for real-time data increases, so does the need for efficient streaming solutions. Technologies like WebSockets and Server-Sent Events (SSE) enable real-time updates, ensuring that charts are always up-to-date without the need for frequent polling or manual refreshes.
Enhanced Browser APIs
Browsers are continually evolving, with new APIs and features being introduced to enhance performance. For example, the WebGPU API provides a more modern and efficient way to leverage GPU acceleration, potentially leading to significant improvements in chart rendering performance.
Integration with Big Data Platforms
Integrating JavaScript charting libraries with big data platforms like Apache Kafka, Apache Flink, and Amazon Kinesis can enable the visualization of massive streams. By leveraging the processing power and scalability of these platforms, developers can create real-time dashboards that handle vast amounts of information with ease.
Practical Tips for Developers
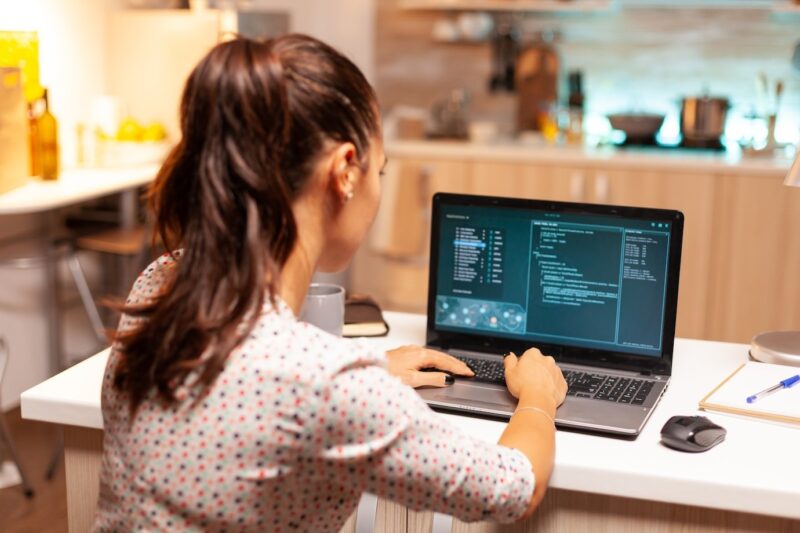
Profiling and Benchmarking
Regular profiling and benchmarking are essential for identifying performance bottlenecks and measuring the impact of optimizations. Tools like Chrome DevTools, Lighthouse, and WebPageTest can provide valuable insights into the performance characteristics of your charts and help you make data-driven decisions.
Code Minification and Bundling
Minifying and bundling your JavaScript code can reduce the size of your scripts, leading to faster load times. Tools like Webpack, Rollup, and Terser can help automate this process, ensuring that your code is optimized for performance.
Avoiding Memory Leaks
Memory leaks can severely impact the performance of your web application, leading to slowdowns and crashes. Ensuring that event listeners are properly removed, and that unused objects are cleaned up, can help prevent memory leaks and maintain smooth performance.
Leveraging CDN for Library Hosting
Hosting your charting libraries on a Content Delivery Network (CDN) can improve load times by reducing the distance between your users and the server. CDNs also offer caching and redundancy, further enhancing the reliability and performance of your web application.
Conclusion
Accelerating JavaScript chart rendering is a multifaceted challenge that requires a combination of efficient fetching, processing, and rendering techniques. By implementing the strategies and techniques discussed in this article, developers can create fast, responsive, and scalable visualizations that meet the demands of modern web applications.
As technology continues to advance, informed about new trends and innovations will be crucial for maintaining optimal performance in your visualizations.